In this post I want to explain how to insert encrypted password while registration and accessing the same with login time. I had implement this at labs.9lessons.info login page. I'm just storing encrypted user password in database. Demo username ='test' and password = 'test'
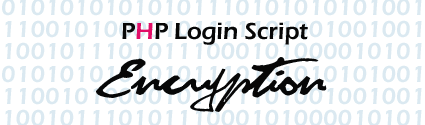


Database
MySQL admin table columns id, username, passcode.
CREATE TABLE admin
(
id INT PRIMARY KEY AUTO_INCREMENT,
username VARCHAR(50) UNIQUE,
passcode VARCHAR(50)
);
(
id INT PRIMARY KEY AUTO_INCREMENT,
username VARCHAR(50) UNIQUE,
passcode VARCHAR(50)
);
Encrypted Password
Here database table admin password:test encrypted and storing like this

registration.php
Contains PHP and HTML code. Just inserting form values into database table admin
<?php
include("db.php");if($_SERVER["REQUEST_METHOD"] == "POST")
{
// username and password sent from Form
$username=mysql_real_escape_string($_POST['username']); $password=mysql_real_escape_string($_POST['password']);
$password=md5($password); // Encrypted Password
$sql="Insert into admin(username,passcode) values('$username','$password');";
$result=mysql_query($sql);
echo "Registration Successfully";
}
?>
<form action="registration.php" method="post">
<label>UserName :</label>
<input type="text" name="username"/><br />
<label>Password :</label>
<input type="password" name="password"/><br/>
<input type="submit" value=" Registration "/><br />
</form>
login.php
Login Script accessing the encrypted password.include("db.php");
session_start();
if($_SERVER["REQUEST_METHOD"] == "POST")
{
$password=mysql_real_escape_string($_POST['password']);
$password=md5($password); // Encrypted Password
$sql="SELECT id FROM admin WHERE username='$username' and passcode='$password'";
$result=mysql_query($sql);
$count=mysql_num_rows($result);
// If result matched $username and $password, table row must be 1 row
if($count==1)
{
header("location: welcome.php");
}
else
{
$error="Your Login Name or Password is invalid";
}
}
if($_SERVER["REQUEST_METHOD"] == "POST")
{
// username and password sent from Form
$username=mysql_real_escape_string($_POST['username']); $password=mysql_real_escape_string($_POST['password']);
$password=md5($password); // Encrypted Password
$sql="SELECT id FROM admin WHERE username='$username' and passcode='$password'";
$result=mysql_query($sql);
$count=mysql_num_rows($result);
// If result matched $username and $password, table row must be 1 row
if($count==1)
{
header("location: welcome.php");
}
else
{
$error="Your Login Name or Password is invalid";
}
}
?>
<form action="login.php" method="post">
<label>UserName :</label>
<input type="text" name="username"/><br />
<label>Password :</label>
<input type="password" name="password"/><br/>
<input type="submit" value=" Login "/><br />
</form>
db.php
Database configuration file.
<?php
$mysql_hostname = "hostname";$mysql_user = "username";
$mysql_password = "password";
$mysql_database = "database";
$bd = mysql_connect($mysql_hostname, $mysql_user, $mysql_password)
or die("Opps some thing went wrong");
mysql_select_db($mysql_database, $bd) or die("Opps some thing went wrong");
?>
The testking 642-845 php tutorials and testking 642-982 live demos are definitely good source of learning especially for php learners. Download the testking EX0-101 tutorial to learn about php login script.
0 comments:
Post a Comment